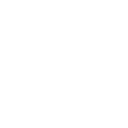
+1
circular dependency in state patrern
In the structure,context refers to state while the state also refers to the context owning it.
Similarly, in the pseudo code, the player owns the state but at the same time the state also refers to the player?
Could you help me to understand is this the right composition? and how does the gabage collection work here? (I know in some modern like swift can let you annoate the order but that does not mean we should have circular dependency here)
Customer support service by UserEcho
I struggle with the same thing, but I think the key is to write to interfaces and not to implementations. I'm not sure what programming language you're using, but in Python it would something like this:
Then, you can use your implementation to work with these interfaces:
Does this help you?